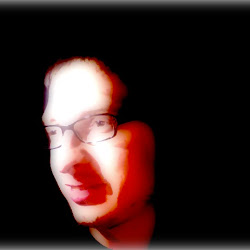
- Johannes Barop <jb@barop.de>
- Hamburg, Germany
- Freelancing web application developer
- Java and GWT
- Game development for fun
Demo Source: savagelook.com
Implement the Game inferface using the core API
import static playn.core.PlayN.*; public class MyGame implements playn.core.Game { void init() { /** ... **/ playn.core.ImageLayer bg = graphics().createImageLayer(bgImage); graphics().rootLayer().add(bg); } /** ... **/ }
Each platform implements the core API
class playn.html.HtmlImageLayer implements playn.core.ImageLayer { /** ... **/ }
Create a project
mvn archetype:generate \ -DarchetypeGroupId=com.googlecode.playn \ -DarchetypeArtifactId=playn-archetype \ -DarchetypeVersion=1.4
Run it
mvn test -Pjava # run it with java mvn test -Phtml # run it with gwt
Android and iOS work too but need some setup.
See code.google.com/p/playn/w for details.
public class MyGame implements Game { @Override public void init() { Image bgImage = assets().getImage("images/bg.png"); ImageLayer bgLayer = graphics().createImageLayer(bgImage); graphics().rootLayer().add(bgLayer); } @Override public void paint(final float alpha) { } @Override public void update(final float delta) { } @Override public int updateRate() { return 25; } }
@Override public void init() { /** **/ Image cloudImage = assets().getImage("images/cloud.png"); ImageLayer cloudLayer = graphics().createImageLayer(cloudImage); graphics().rootLayer().add(cloudLayer); }
@Override public void paint(final float delta) { couldX += delta; if (couldX > bgImage.width() - cloudImage.width()) { couldX = -cloudImage.width(); } cloudLayer.setTranslation(couldX, couldY); }
@Override public void init() { /** **/ ballImage = assets().getImage("images/ball.png"); ballLayer = graphics().createGroupLayer(); graphics().rootLayer().add(ballLayer); pointer().setListener(new Pointer.Adapter() { @Override public void onPointerEnd(final Pointer.Event event) { Ball ball = new Ball(ballImage, event.x(), event.y()); ballLayer.add(ball.layer); } }); }
public Ball(final Image image, final float x, final float y) { /** **/ BodyDef bodyDef = new BodyDef(); bodyDef.type = BodyType.DYNAMIC; bodyDef.position = new Vec2(x, y); body = world.createBody(bodyDef); FixtureDef fixtureDef = new FixtureDef(); fixtureDef.shape = new CircleShape(); fixtureDef.shape.m_radius = radius; fixtureDef.density = 1.0f; fixtureDef.friction = 0.3f; body.createFixture(fixtureDef); body.setTransform(new Vec2(x, y), 0.0f); }
@Override public void init() { /** **/ world = new World(new Vec2(0.0f, 10.0f), true); world.setWarmStarting(true); world.setAutoClearForces(true); } @Override public void update(final float delta) { world.step(delta, 10, 10); }
@Override public void init() { /** **/ float physWidth = physUnitPerScreenUnit * 640; float physHeight = physUnitPerScreenUnit * 480; Body ground = world.createBody(new BodyDef()); PolygonShape bottom = new PolygonShape(); bottom.setAsEdge(new Vec2(0, physHeight), new Vec2(physWidth, physHeight)); ground.createFixture(bottom, 0.0f); PolygonShape top = new PolygonShape(); top.setAsEdge(new Vec2(0, 0), new Vec2(physWidth, 0)); ground.createFixture(top, 0.0f); PolygonShape left = new PolygonShape(); left.setAsEdge(new Vec2(0, 0), new Vec2(0, physHeight)); ground.createFixture(left, 0.0f); PolygonShape right = new PolygonShape(); right.setAsEdge(new Vec2(physWidth, 0), new Vec2(physWidth, physHeight)); ground.createFixture(right, 0.0f); }
Please give feedback